Ruby on Rails is a young, lean framework focused on developer productivity and happiness. The chief reason for Rails’s growing popularity is the ability to rapidly build, enhance, and scale websites and web services thanks to its cutting-edge design, human-readable syntax, and open ethos of sharing your ideas with others. Working in Rails makes you part of a larger community of forward-thinking programmers advancing the vanguard of web technology.
This book focuses on Rails fundamentals, including a primer on the Ruby programming language. A strong emphasis is placed on best practices and the Model-View-Controller (MVC) paradigm. By the end of the first chapter, you’ll have built a fully-functioning Rails website, and by the end of the book, you will have had a tour through the major components of Rails.
Includes Downloadable Class Files (works on Mac)
ISBN: 978-1-934624-43-2
Setup & Introduction
Downloading the Class Files
Before You Begin: Installing Ruby on Rails
- Installing Command Line Tools
- Installing Homebrew
- Installing Ruby Version Manager (RVM)
- Installing Ruby & Rails
Section 1
Setting Up: Do This Before Other Exercises!
- Setting up your class files
Scaffolding
- Getting started
- Generating a scaffold
- Adding content
Adjusting the Templates Created by Scaffolding
- Formatting Rails
- Coding simple styles in Rails
- Coding title bar titles
- Redirecting the site root page
- Editing the CSS
- Adding basic security
Ruby Fundamentals: Classes & Objects
- Everything in Ruby is an object
- Defining a class
Section 2
Ruby Fundamentals: Properties & Variables
- Properties of objects
- Instance variables & local variables
- Global variables
Ruby Fundamentals: Manipulating Variables
- Creating strings in Ruby
- Simple string methods: changing case
- Substrings
- Ranges
- Comparing strings
- Regular expressions
Ruby: Sanitizing User Input & Control Structures
- Sanitizing user input
- Integers & decimals
- If/else, unless, & case statements
- Constants
- Symbols
Ruby: Collections
- Arrays: the simplest collections
- Hashes
- Enumerators
- Common iterators
Section 3
MVC: Creating a Model, View, & Controller
- MVC: model-view-controller
- Creating a new Rails site for Flix
- Generating a new model
- Editing a migration file
- Populating a database with a seed file
- Creating a new controller
Integrating the Front-End Designer’s Code
- Incorporating the designer’s HTML & CSS
- Incorporating the JavaScript files, images, & fonts
MVC: Controllers & Routing
- Resourceful vs. non-resourceful routing
- Assigning instance variables
- What if the names don’t match?
- Optional bonus: redirects
MVC: Views
- Creating a view
- Adding dynamic data
- Rendering a partial
- Optional bonus: rendering a view
Section 4
Forms in Rails: Creating the Form
- form_tag & form_for
- Checkboxes, radio buttons, & select boxes
- Adding a dropdown menu
- Adding a date selector & submit button
Forms in Rails: Processing & Editing Form Data
- Making the form work: defining a create method
- Making an edit form
- Optional bonus: DRYing up the code even more
Model Creation & Management
- Generating & rolling back a migration
- Updating views & controllers to match an updated model
- Viewing the contents of a database
Exploring & Validating Models
- Exploring database contents in Rails console
- Adding an object in Rails console
- Editing an object in Rails console
- Adding basic validation to a model
Section 5
Model Methods & Scopes
- Creating a model method for runtime
- Scopes
- Optional bonus: DRYing up the scopes
- Additional bonus: adding the tab highlight behavior
Model Relationships
- Creating a model for cast members
- Adding objects to the cast members model in Rails Console
- Updating views to include the cast members model
- Creating a genre model
- Adding a genre field to the edit form
Model Relationships: Creating a Genre View
- Creating a genre controller
- Creating a genre view
- Improving the look of the genre view
Section 6
Introduction to Testing
- Fixtures
- Basic tests: assert & refute
- Other assert methods
- The importance of error messages
- Writing simple tests using fixtures
- Optional bonus: writing tests using embedded Ruby code
- Additional bonus: helpers
MVC Tests
- What to test
- Testing a custom validation
- Testing a model method
- Testing a controller
Gems: Plugins for Ruby
- What is a gem?
- Gemfile and Gemfile.lock
- Installing a new gem
- Adding sign in & sign out links
- Adding basic user authentication
- Removing the ability for users to register themselves
Bonus Material
Managing Your Code with Git
- Creating a new Git repository
- Tracking changes & adding files
- Committing code to Git
- GitHub: pushing your code to the cloud
- Committing a change to the repository
- Cloning a repository
Reference Material
Basic Structure of Scaffolding
Examples of What You Learn
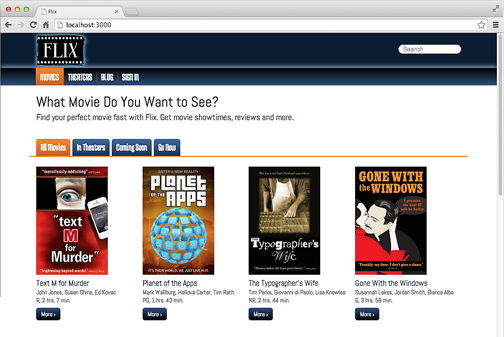
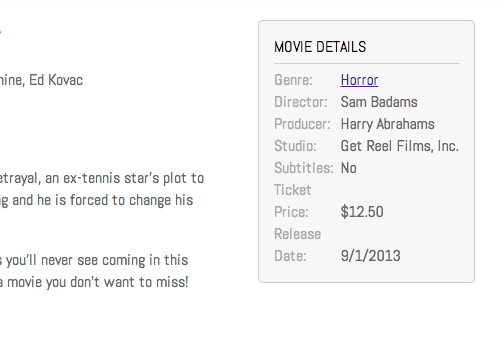
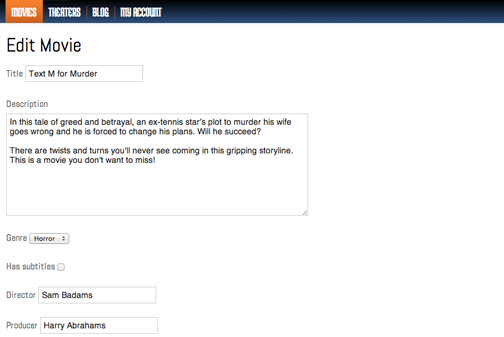
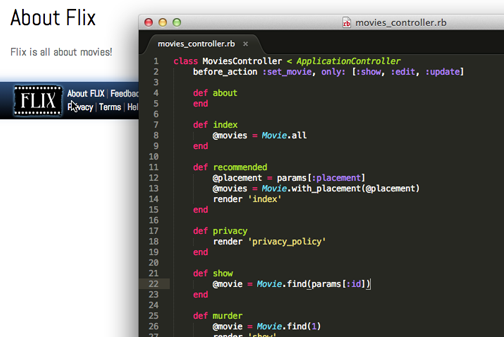
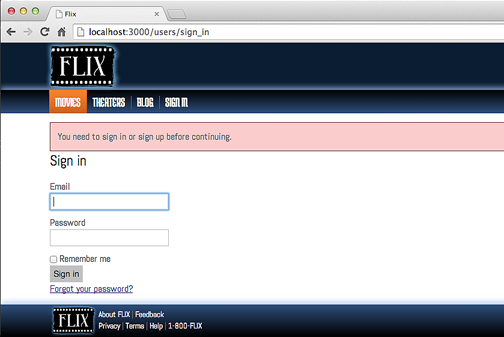
Why Our Books Are Unique
Our books are packed with step-by-step exercises that walk you through projects. You’ll learn by doing exercises, not reading long explanations. The goal is to give you hands-on practice with the program, getting you started quickly with the things that are most important for real life work.