As the app gold rush continues iOS development is high in demand. This book is designed to get your Objective-C programming skills up to par as a precursor to our Intro to iPhone/iPad Development book. You'll be guided through the basics of C and Objective-C programming as it relates to iOS—all via Apple's XCode software. It is an entry into the world of iOS development.
Objective-C is a powerful, object-oriented extension to the C programming language. This training is geared towards the beginning programming student and covers basic syntax and programming concepts. No prior programming knowledge required.
Includes Downloadable Class Files (works on Mac)
ISBN: 978-1-934624-44-9
Section 1
Setting Up For Class: Do This Exercise First!
- Setting Up Class Files
The C Programming Language
- The Xcode Workspace
- Outputting to the Console
- The printf() Function
- Comments
Variables
- Data Types
- Declaring and Updating Variables
- Format Specifiers
- Floats and Booleans
- Arithmetic Operators
Conditional Statements & Operators
- The if Statement
- The else Statement
- Comparison Operators
- The else if Statement
- Logical Operators
Loops & Arrays
- The for Loop
- The while Loop
- C-Style Arrays
- Iterating over Arrays
Section 2
Creating a Class
- Object-Oriented Programming
- Objects and Classes
- Header and Implementation Files
- Defining Methods
- The NSLog() Function
- Instantiation
- Messaging
Properties
- Declaring Properties
- Primitive Data Types
- Creating Properties using Objects
- Pointers
Methods
- Methods with Parameters
- Methods with Return Types
- Class Methods
Instantiation
- Instantiating Multiple Objects
- Using Apple-Provided Classes
Section 3
Inheritance
- Creating a Subclass
- Method Overriding
Categories
- Extending Existing Classes
- Importing Categories
Protocol and Delegates
- Defining and Implementing Protocol
- Implementing Protocols
- UIAlertViewDelegate
Blocks
- Defining a Block
- Blocks with Parameters
- Blocks with Return Types
- Completion Blocks (Callbacks)
Object-Oriented Primitives
- The id Data Type
- The Class Data Type
- The SEL Data Type
Section 4
NSString
- Objective-C Literals
- Comparing Two Strings
- Concatenating Strings
NSNumber
- numberWithInt, numberWithFloat, and numberWithBool
- NSNumber Literals
- isEqualToNumber
- intValue, floatValue, boolValue, and stringValue
NSArray & NSMutableArray
- NSArray Literals
- Updating Values in an NSMutableArray
- Fast Enumeration
NSDictionary & NSMutableDictionary
- NSDictionary Literals
- Key-Value Pairs
- Updating Values in an NSMutableDictionary
- Removing Key-Value Pairs
User Login Exercise
- Creating a User Object
- Storing Users in an NSMutableArray
- Creating a Log-In Method
Reference Material
Automatic Reference Counting
Examples of What You Learn
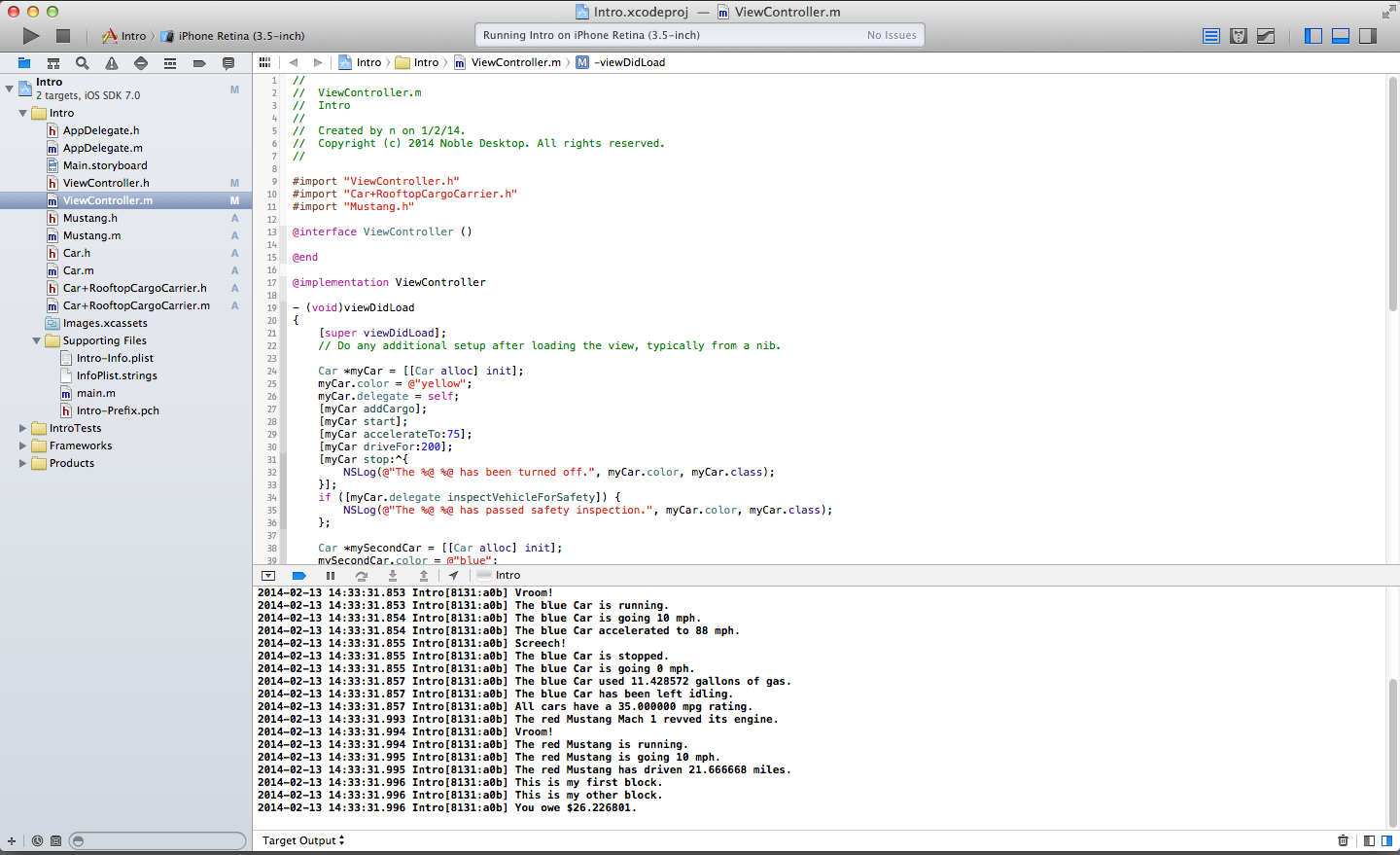
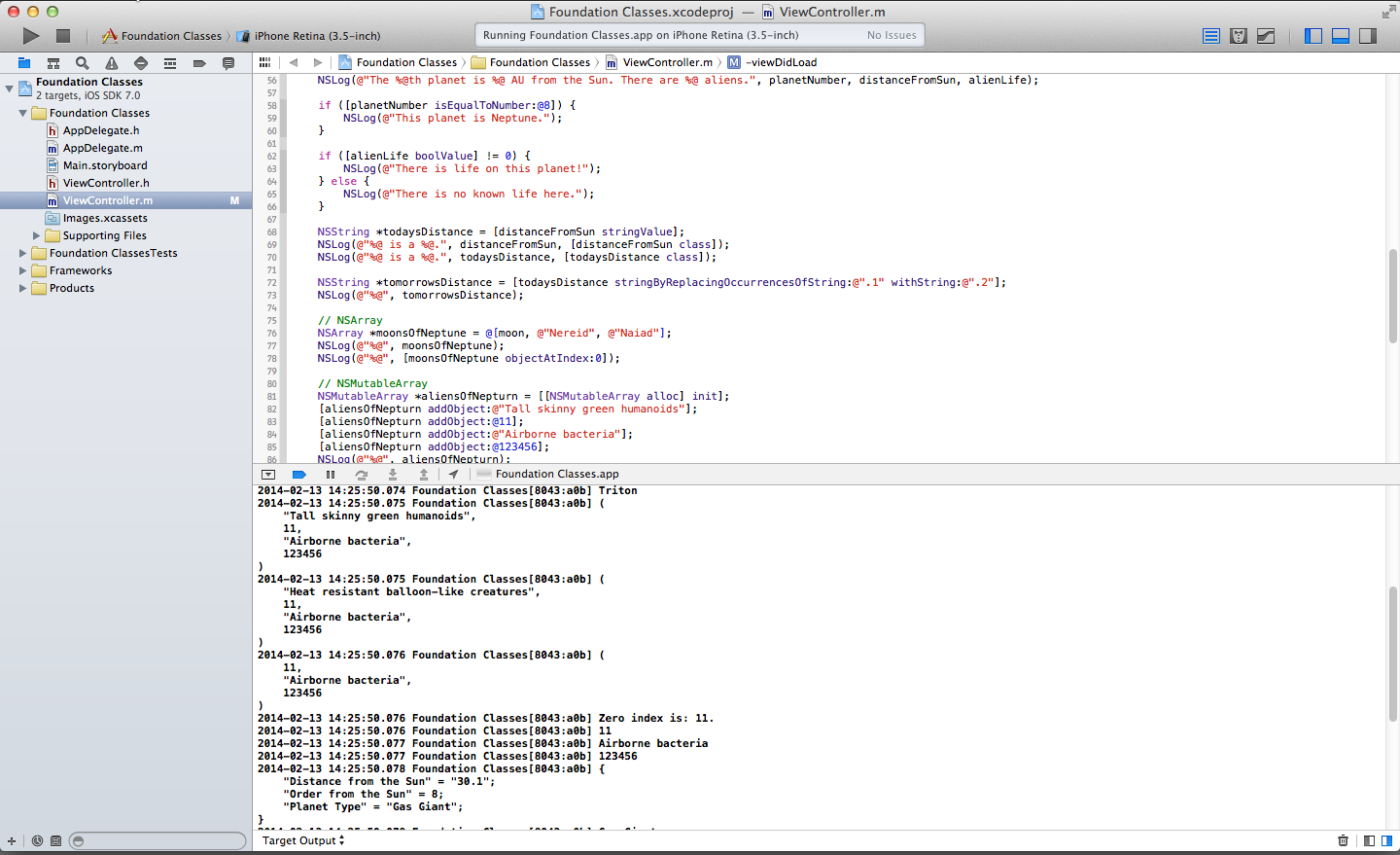
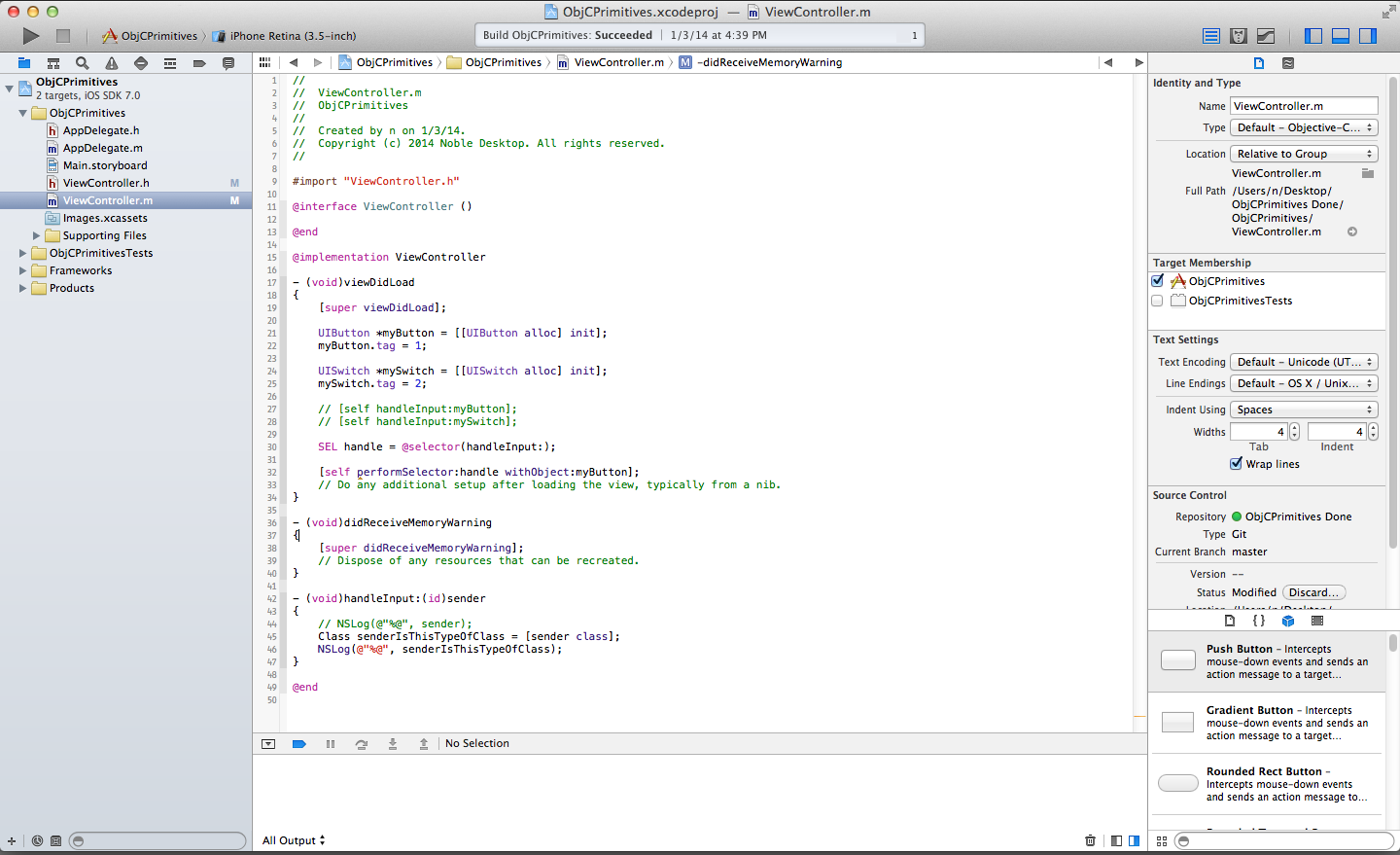
Why Our Books Are Unique
Our books are packed with step-by-step exercises that walk you through projects. You’ll learn by doing exercises, not reading long explanations. The goal is to give you hands-on practice with the program, getting you started quickly with the things that are most important for real life work.